Time to market is critical for a digital product’s success: You should deliver it as fast as possible to win customers from competitors. One of the productivity boosters widely used in software development is object-relational mapping (ORM) — an effective way to establish seamless communication between structures in an app and a relational database. This article explains why ORM is important, what mapping tools exist, and when it’s better to avoid this approach — no matter how beneficial it may seem.
What is object-relational mapping?
Object-relational mapping (ORM) is an abstraction layer that simplifies interactions between two different data representations— tables in relational databases and objects constructed with object-oriented programming (OOP) languages, such as C++, Java, Ruby, C#, PHP, Python, TypeScript, and many others.
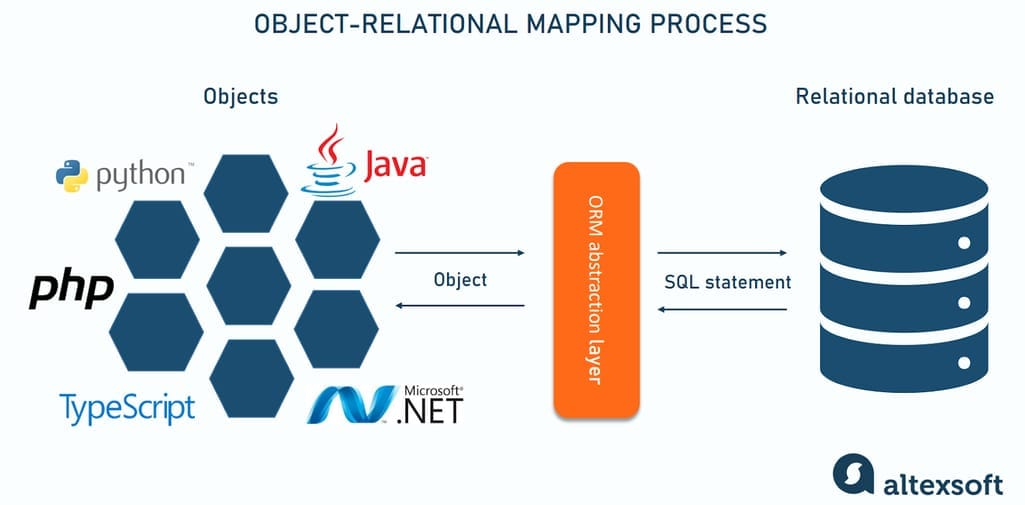
ORM abstraction layer between objects in OOP code and tables in a relational database
In OOP, an object is a combination of data (attributes) and program logic (behavior or what to do with the data) that corresponds to real-world instances — for example, a car, a person, or a bank transaction. Objects with similar behavior and attributes are grouped into classes.
For example, in a flight booking system, there can be a flight class where an object represents a particular flight and contains information on its number, route, and schedule. The behavior part may dictate how to check seat availability and retrieve a seat map when a passenger reserves air travel.
Typically, developers have to employ SQL (structured query language) to save app data in relational databases as well as to modify and retrieve it. ORM creates a map describing relationships between objects and tables, abstracting away the details of how data is organized. It automatically generates SQL queries so engineers can continue using structures native to the programming language of their choice.
ORM advantages
Besides sparing developers from learning SQL, object-oriented mapping brings other significant benefits.
Database independence. ORM enables you to apply the same code across different relational database management systems (DBMSs). As a result, businesses switch between DBMSs with lower investments in time and money. In turn, developers may share the code and reuse it to test how the program works with various databases.
Faster development. With ORM, engineers omit writing boilerplate SQL commands and can reuse the code multiple times. As a result, they become more productive, especially at the start of the project, having more time to focus on business logic rather than on building queries.
Simpler code writing and maintenance. Since you don’t need to write SQL queries inside the code, it becomes more readable, clear, and thus easier to maintain.
Enhanced security. ORM systems have embedded safeguards against SQL injection attacks — a common malicious activity when a hacker sends malicious queries to make the app execute unwanted commands.
Advanced features. Many ORM tools come with extensive functionality that makes developers even more productive. Among the most useful features are automatic database schema migration and query optimization.
ORM drawbacks
ORM is certainly not a one-size-fits-all solution. We’ve made a list of the most often mentioned pitfalls to consider.
Problems with debugging and query optimization. The abstraction layer ensures one of the biggest ORM advantages — the ability to use the same code for different databases. But there’s a trade-off: Developers have little-to-no control over how queries are generated and how the app interacts with the database under the hood. This poses specific challenges when it comes to identifying and removing low-level errors. There is also no way to optimize queries.
Lower app speed and performance. Because of the abstraction layer and related extra operations, the software can run slower with ORM than with raw SQL. This is particularly true when an app works with complex queries against large datasets containing millions of records.
Vendor lock-in. Though ORM grants you independence from databases, it, at the same time, ties you to a particular library, framework, or API provider. Sometimes, developers find it difficult to move to other solutions.
Steep learning curve. Freeing you from mastering SQL, ORM by itself is not the most straightforward technology to comprehend. The learning curve can be particularly hard for beginner developers who are vaguely familiar with OOP concepts. Also, you still need to get familiar with a specific query language used by a particular mapper.
If, after weighing all pros and cons, you decide to give ORM a try, it’s time to look at solutions available in the market and what they can really do.
ORM tools overview
Each OOP programming language has its own ORM tools to perform object-relational mapping operations. We’ll focus on the most popular technologies and related ORM solutions.
Java ORM: Hibernate, EclipseLink, and OpenJPA
ORM tools for Java employ Jakarta Persistence API (JPA) specification, where persistence means an ability to save app information in a long-lasting storage medium and retrieve it when necessary. JPA defines how to access relational DBMS from Java, store Java objects, and handle relationships between different database tables within one system. Here are several popular JPA implementations.
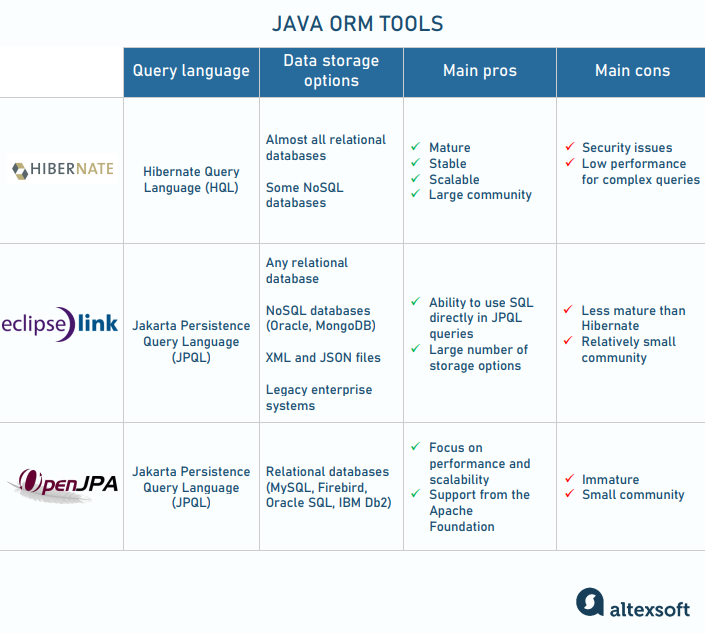
Most popular ORM tools for Java development
Hibernate is one of the most mature and widely used ORM tools, compatible with many popular Java frameworks and supported by a large community. Developers appreciate its open-source nature, stability, and scalability.
It also boasts relatively high performance achieved due to the embedded caching mechanism that stores the most frequently accessed data. While Hibernate handles queries of any complexity, it’s better to simplify table structures since large commands can slow down the app.
The lightweight solution uses Hibernate Query Language (HQL), which is SQL-like but works with Java objects and supports almost all relational databases. Some extensions even let you employ the ORM tool for NoSQL systems as well. In addition to HQL, engineers can also use an SQL version, native to a particular database.
EclipseLink is another popular open-source ORM tool for Java. Besides JPA, it supports other standards to link Java to other data storage options — such as
- XML documents,
- JSON documents,
- legacy enterprise systems, and more.
EclipseLink also enables you to interact with non-relational databases — namely, Oracle NoSQL and MongoDB — via the JPA connection. The instrument relies on Jakarta Persistence Query Language (JPQL), which is platform-agnostic, object-oriented, and inherent to JPA. Resembling SQL in syntax, JPQL allows the use of SQL commands right in its queries (in Hibernate, it’s not directly possible.)
Note that EclipseLink is tightly interconnected with the Oracle ecosystem, sharing a database with its proprietary ORM tool — TopLink. So, it’s a number one choice for projects that utilize many Oracle products and services.
OpenJPA is an open-source project by the Apache Foundation. Similar to EclipseLink, it uses a project-oriented JPQL language to query SQL databases.
The tool is known for its focus on performance and scalability. It also provides a toolset for testing your app components against different databases — it’s better to use Docker containers for this purpose. At the same time, OpenJPA is the youngest and the least mature of the three mentioned Java ORM options.
Python ORM: Django, SQLAlchemy, Peewee, and Pony ORM
ORM tools in the Python ecosystem allow you to build queries using Pythonic syntax. Below, we list only the four most popular open-source options, but there are more of them worth considering.
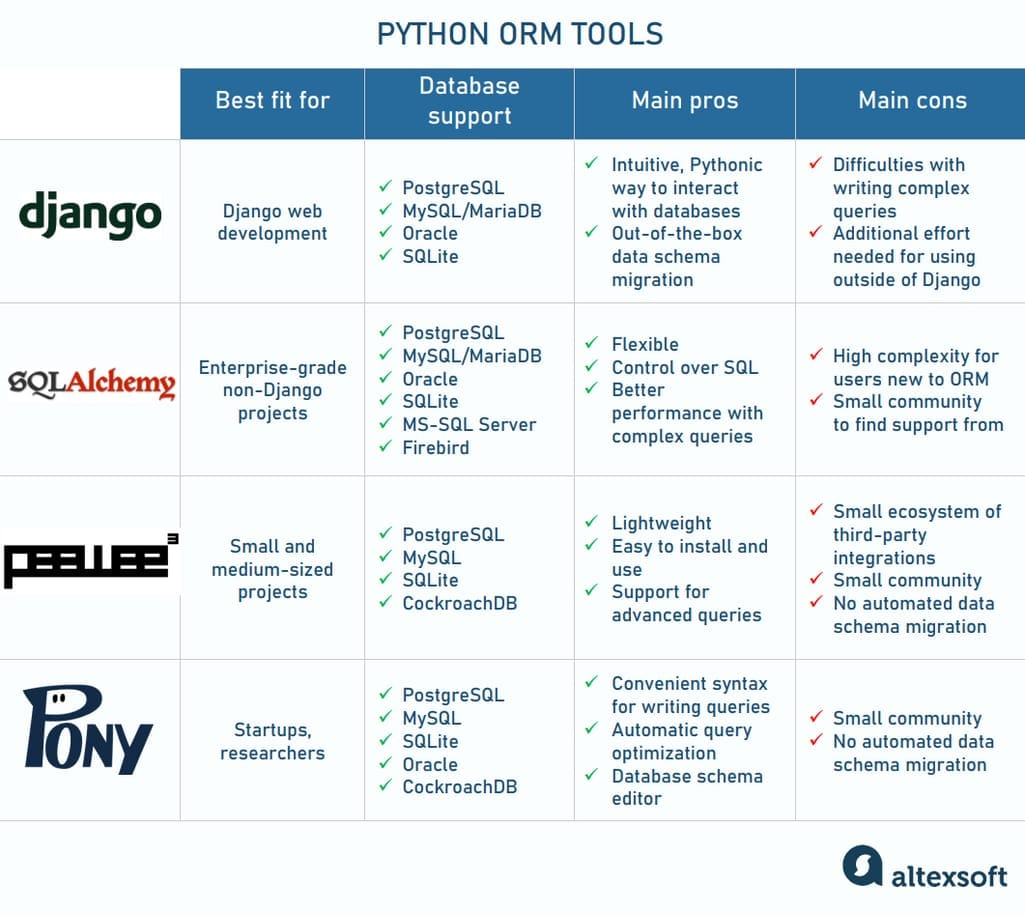
Most popular ORM tools in Python ecosystem
Django ORM is a module embedded into the web framework of the same name. It provides a straightforward way to interact with a range of SQL databases using Python. In addition, it automatically writes scripts for data migration when you move from one schema to another.
At the same time, developers often note that it’s easier to build complex queries with SQL or other ORM options than with the Django module. If you still want to try it as a standalone objective-related mapper, you have to load and set up other parts of Django.
SQLAlchemy gained popularity as the gold standard for ORM in Python due to its flexibility and total control over query structure. The tool has two interfaces to communicate with databases — the Core, which is closer to raw SQL, and the ORM. The Core enables building complex Pythonic queries that render directly in SQL, so you can achieve better performance when working with large databases.
In turn, the ORM creates an additional layer of abstraction to map Python classes into a database schema and vice versa.
Peewee is a lightweight and easy-to-use ORM library. Its focus on simplicity makes the tool a popular choice for small and medium-sized projects. At the same time, Peewee supports complex query operations that allow developers to build data-driven business apps.
As for cons, Peewee interacts with fewer databases than Django ORM and SQLAlchemy. It doesn’t automate schema migration, nor does it boast a large community or extensive ecosystem of third-party integrations.
Pony ORM stands out from its competitors thanks to several unique features. They include extremely convenient Python syntax, automatic query optimization, and an online schema editor to create data models and generate database tables from them. It also offers support for JSON data type and a smart caching mechanism for queries and objects. Unfortunately, Pony ORM doesn’t provide tooling for schema migration.
ORM for .NET and C#: Entity Framework Core, Dapper, and NHibernate
There are three powerful ORM tools serving the .NET development framework. Each comes with its advantages and disadvantages fitting different project types.
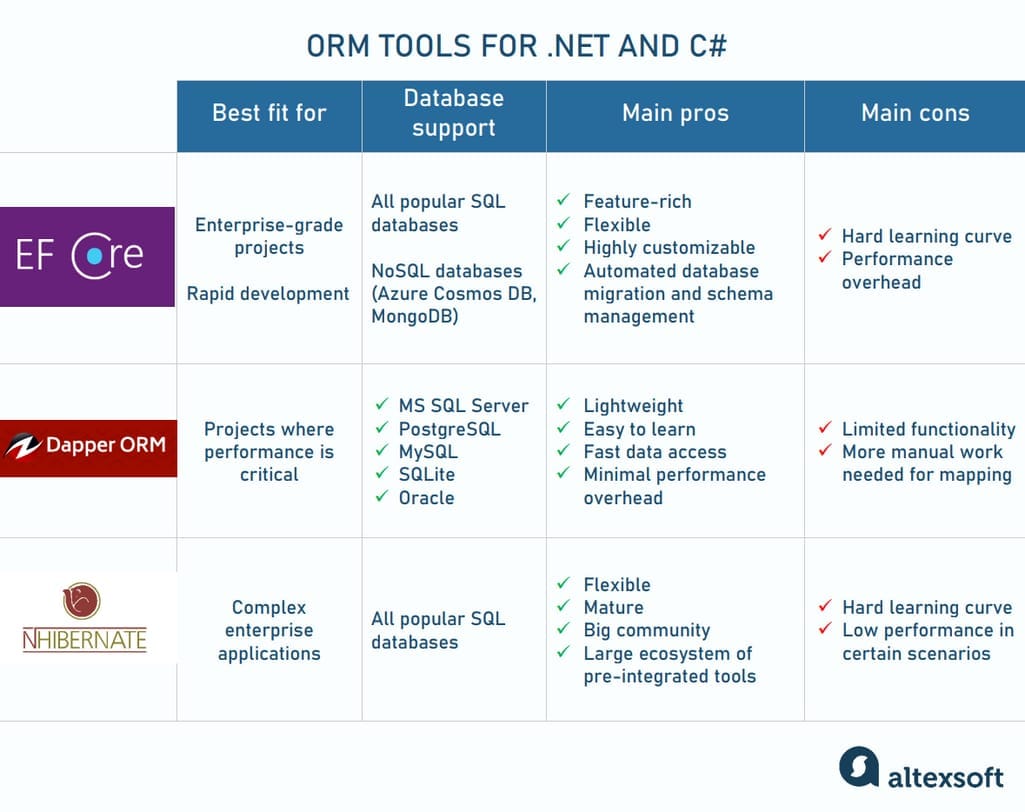
Best ORM tools for .NET developers
Entity Framework Core (EF Core) is an official object-relational mapper for .Net (C#). Developed by Microsoft, it’s natively integrated with other MS technologies. EF Core applies plugins to access a wide range of databases — relational and NoSQL (such as Azure Cosmos DB and MongoDB). It lets you build data schemas using C# classes and create complex queries with LINQ (Language Integrated Query), which is more intuitive for .NET developers than SQL.
The framework also has embedded mechanisms for database migration, change tracking, and schema management. All in all, it’s a powerful, flexible, and highly configurable instrument with strong community support and great documentation. On the dark side, such rich functionality can result in performance overhead and a harder learning curve.
Dapper is a lightweight, easy-to-learn framework with a focus on simplicity and high performance. Unlike other ORM tools, It doesn’t have a database abstraction layer. Instead, it relies on ADO.NET — a technology that simplifies access to various data sources (relational, XML, and others) for .NET developers.
Dapper enables you to transfer raw SQL queries to objects and vice versa with minimal performance overhead and quick execution of database operations. At the same time, mapping with Dapper takes more manual effort and requires knowing SQL. Compared to EF Core, Dapper has fewer features — it doesn’t support database migration or change tracking.
NHibernate (NH) is a mature ORM inspired by Hibernate for Java and extremely popular in enterprise environments. It boasts great flexibility and extensive mapping features for C# and Visual Basic.NET object models, including almost all features of Java’s Hibernate.
You can extend NH functionality even more since it leverages a large ecosystem of pre-integrated commercial and open-source tools. Another great thing about the mapper is its big and active community of supporters. Among key disadvantages mentioned by developers is low performance in certain scenarios (for example, statistical querying systems).
When to use ORM?
So, when should you prefer ORM over SQL? The answer largely depends on your project's complexity, the skills of your development team, specific requirements, and other factors. Here are several typical scenarios that will benefit from ORM.
- Your application is not supposed to conduct complex queries, nor does it require especially high performance.
- High speed of development is your priority.
- You’re building a throwaway prototype — in other words, an early app version that helps gather feedback and clarify requirements but will eventually be discarded.
- Your software is supposed to often change a database schema or have to work with several DBMSs.
- Your team members have deep experience with OOP but are not as proficient with SQL.
It’s worth noting that most ORM solutions — and especially enterprise-grade ones — have different embedded mechanisms to address the problem of relatively low speed and performance. This includes different caching strategies that can decrease the number of database queries. Also, object-relational mappers often support lazy loading that enables the app to fetch only requested data and postpone the load of any related information. This practice reduces memory consumption and spares the system unnecessary database operations.
But despite all the advanced features, it’s still faster to execute SQL queries than to run object-to-table transformations. So, if performance is critical for your app, stick to raw SQL. And use ORM for projects where time to market matters most.